Back
Back
Back
Orb Background
Orb Background
Page Contents
Page Contents
Page Contents



A very cool background effect great for startups.
OrbFrame is a custom Framer component that beautifully enhances "The Orb" from React Bits with additional functionality and design flexibility. This component creates a responsive, interactive frame around The Orb's mesmerizing glowing, particle-driven background.
Preview and Remix >>
Key Features:
Seamless Integration: Works perfectly within Framer's design-first environment
Customizable Properties: Adjust border radius, shadow intensity, and hover animations
Interactive Enhancements: Scale animations triggered on hover create engaging user interactions
Full Compatibility: Syncs with all of The Orb's existing customization options including gradient colors and animation speed
Responsive Design: Adapts beautifully to different screen sizes and containers
Perfect for modern landing pages, dynamic UI mockups, and any Framer project needing that extra visual impact. The component is lightweight and follows a simple drag-and-drop implementation, making it accessible for designers of all skill levels.
Take your Framer designs to the next level with this polished, eye-catching effect that combines the mesmerizing qualities of The Orb with enhanced framing and interaction capabilities.
If you are not sure how to add code to Framer watch this quick video.
Framer Code Component
Orb Background
1.0
1.0
1.0
1.0
// A custom Framer code component by Chris Kellett - Framerverse
// v1.0.0
import React, { useEffect, useRef } from "react"
import { addPropertyControls, ControlType } from "framer"
// OGL is imported directly in this component
// Mini version of OGL focused on what we need for this component
class Vec3 {
constructor(x = 0, y = 0, z = 0) {
this.x = x
this.y = y
this.z = z
}
set(x, y, z) {
this.x = x
this.y = y
this.z = z
return this
}
}
class Renderer {
constructor(options = {}) {
this.gl = document.createElement("canvas").getContext("webgl", {
alpha: options.alpha || false,
premultipliedAlpha: options.premultipliedAlpha || false,
antialias: options.antialias || false,
})
}
setSize(width, height) {
this.gl.canvas.width = width
this.gl.canvas.height = height
this.gl.viewport(0, 0, width, height)
}
render({ scene }) {
scene.draw()
}
}
class Program {
constructor(gl, { vertex, fragment, uniforms = {} }) {
this.gl = gl
this.uniforms = uniforms
const vertexShader = this.createShader(gl.VERTEX_SHADER, vertex)
const fragmentShader = this.createShader(gl.FRAGMENT_SHADER, fragment)
this.program = gl.createProgram()
gl.attachShader(this.program, vertexShader)
gl.attachShader(this.program, fragmentShader)
gl.linkProgram(this.program)
if (!gl.getProgramParameter(this.program, gl.LINK_STATUS)) {
console.error(
"Failed to link program:",
gl.getProgramInfoLog(this.program)
)
gl.deleteProgram(this.program)
return null
}
// Initialize uniform locations
this.uniformLocations = {}
for (const name in uniforms) {
this.uniformLocations[name] = gl.getUniformLocation(
this.program,
name
)
}
// Initialize attribute locations
this.attributeLocations = {
position: gl.getAttribLocation(this.program, "position"),
uv: gl.getAttribLocation(this.program, "uv"),
}
}
createShader(type, source) {
const gl = this.gl
const shader = gl.createShader(type)
gl.shaderSource(shader, source)
gl.compileShader(shader)
if (!gl.getShaderParameter(shader, gl.COMPILE_STATUS)) {
console.error(
"Failed to compile shader:",
gl.getShaderInfoLog(shader)
)
gl.deleteShader(shader)
return null
}
return shader
}
use() {
this.gl.useProgram(this.program)
this.setUniforms()
return this
}
setUniforms() {
const gl = this.gl
for (const name in this.uniforms) {
const value = this.uniforms[name].value
const location = this.uniformLocations[name]
if (typeof value === "number") {
gl.uniform1f(location, value)
} else if (value instanceof Vec3) {
gl.uniform3f(location, value.x, value.y, value.z)
}
}
}
}
class Triangle {
constructor(gl) {
this.gl = gl
// Define triangle vertices
const vertices = new Float32Array([-1, -1, 3, -1, -1, 3])
// Define UVs
const uvs = new Float32Array([0, 0, 2, 0, 0, 2])
// Create buffers
this.positionBuffer = gl.createBuffer()
gl.bindBuffer(gl.ARRAY_BUFFER, this.positionBuffer)
gl.bufferData(gl.ARRAY_BUFFER, vertices, gl.STATIC_DRAW)
this.uvBuffer = gl.createBuffer()
gl.bindBuffer(gl.ARRAY_BUFFER, this.uvBuffer)
gl.bufferData(gl.ARRAY_BUFFER, uvs, gl.STATIC_DRAW)
}
bind(program) {
const gl = this.gl
gl.bindBuffer(gl.ARRAY_BUFFER, this.positionBuffer)
gl.enableVertexAttribArray(program.attributeLocations.position)
gl.vertexAttribPointer(
program.attributeLocations.position,
2,
gl.FLOAT,
false,
0,
0
)
gl.bindBuffer(gl.ARRAY_BUFFER, this.uvBuffer)
gl.enableVertexAttribArray(program.attributeLocations.uv)
gl.vertexAttribPointer(
program.attributeLocations.uv,
2,
gl.FLOAT,
false,
0,
0
)
}
}
class Mesh {
constructor(gl, { geometry, program }) {
this.gl = gl
this.geometry = geometry
this.program = program
}
draw() {
const gl = this.gl
this.program.use()
this.geometry.bind(this.program)
gl.drawArrays(gl.TRIANGLES, 0, 3)
}
}
// Main Orb component
export default function Orb({
hue = 0,
hoverIntensity = 0.2,
rotateOnHover = true,
forceHoverState = false,
width = "100%",
height = "100%",
}) {
const ctnDom = useRef(null)
// Vertex shader code
const vert = /* glsl */ `
precision highp float;
attribute vec2 position;
attribute vec2 uv;
varying vec2 vUv;
void main() {
vUv = uv;
gl_Position = vec4(position, 0.0, 1.0);
}
`
// Fragment shader code
const frag = /* glsl */ `
precision highp float;
uniform float iTime;
uniform vec3 iResolution;
uniform float hue;
uniform float hover;
uniform float rot;
uniform float hoverIntensity;
varying vec2 vUv;
vec3 rgb2yiq(vec3 c) {
float y = dot(c, vec3(0.299, 0.587, 0.114));
float i = dot(c, vec3(0.596, -0.274, -0.322));
float q = dot(c, vec3(0.211, -0.523, 0.312));
return vec3(y, i, q);
}
vec3 yiq2rgb(vec3 c) {
float r = c.x + 0.956 * c.y + 0.621 * c.z;
float g = c.x - 0.272 * c.y - 0.647 * c.z;
float b = c.x - 1.106 * c.y + 1.703 * c.z;
return vec3(r, g, b);
}
vec3 adjustHue(vec3 color, float hueDeg) {
float hueRad = hueDeg * 3.14159265 / 180.0;
vec3 yiq = rgb2yiq(color);
float cosA = cos(hueRad);
float sinA = sin(hueRad);
float i = yiq.y * cosA - yiq.z * sinA;
float q = yiq.y * sinA + yiq.z * cosA;
yiq.y = i;
yiq.z = q;
return yiq2rgb(yiq);
}
vec3 hash33(vec3 p3) {
p3 = fract(p3 * vec3(0.1031, 0.11369, 0.13787));
p3 += dot(p3, p3.yxz + 19.19);
return -1.0 + 2.0 * fract(vec3(
p3.x + p3.y,
p3.x + p3.z,
p3.y + p3.z
) * p3.zyx);
}
float snoise3(vec3 p) {
const float K1 = 0.333333333;
const float K2 = 0.166666667;
vec3 i = floor(p + (p.x + p.y + p.z) * K1);
vec3 d0 = p - (i - (i.x + i.y + i.z) * K2);
vec3 e = step(vec3(0.0), d0 - d0.yzx);
vec3 i1 = e * (1.0 - e.zxy);
vec3 i2 = 1.0 - e.zxy * (1.0 - e);
vec3 d1 = d0 - (i1 - K2);
vec3 d2 = d0 - (i2 - K1);
vec3 d3 = d0 - 0.5;
vec4 h = max(0.6 - vec4(
dot(d0, d0),
dot(d1, d1),
dot(d2, d2),
dot(d3, d3)
), 0.0);
vec4 n = h * h * h * h * vec4(
dot(d0, hash33(i)),
dot(d1, hash33(i + i1)),
dot(d2, hash33(i + i2)),
dot(d3, hash33(i + 1.0))
);
return dot(vec4(31.316), n);
}
// Instead of "extractAlpha" that normalizes the color,
// we keep the computed color as-is and later multiply by alpha.
vec4 extractAlpha(vec3 colorIn) {
float a = max(max(colorIn.r, colorIn.g), colorIn.b);
return vec4(colorIn.rgb / (a + 1e-5), a);
}
const vec3 baseColor1 = vec3(0.611765, 0.262745, 0.996078);
const vec3 baseColor2 = vec3(0.298039, 0.760784, 0.913725);
const vec3 baseColor3 = vec3(0.062745, 0.078431, 0.600000);
const float innerRadius = 0.6;
const float noiseScale = 0.65;
float light1(float intensity, float attenuation, float dist) {
return intensity / (1.0 + dist * attenuation);
}
float light2(float intensity, float attenuation, float dist) {
return intensity / (1.0 + dist * dist * attenuation);
}
vec4 draw(vec2 uv) {
vec3 color1 = adjustHue(baseColor1, hue);
vec3 color2 = adjustHue(baseColor2, hue);
vec3 color3 = adjustHue(baseColor3, hue);
float ang = atan(uv.y, uv.x);
float len = length(uv);
float invLen = len > 0.0 ? 1.0 / len : 0.0;
float n0 = snoise3(vec3(uv * noiseScale, iTime * 0.5)) * 0.5 + 0.5;
float r0 = mix(mix(innerRadius, 1.0, 0.4), mix(innerRadius, 1.0, 0.6), n0);
float d0 = distance(uv, (r0 * invLen) * uv);
float v0 = light1(1.0, 10.0, d0);
v0 *= smoothstep(r0 * 1.05, r0, len);
float cl = cos(ang + iTime * 2.0) * 0.5 + 0.5;
float a = iTime * -1.0;
vec2 pos = vec2(cos(a), sin(a)) * r0;
float d = distance(uv, pos);
float v1 = light2(1.5, 5.0, d);
v1 *= light1(1.0, 50.0, d0);
float v2 = smoothstep(1.0, mix(innerRadius, 1.0, n0 * 0.5), len);
float v3 = smoothstep(innerRadius, mix(innerRadius, 1.0, 0.5), len);
vec3 col = mix(color1, color2, cl);
col = mix(color3, col, v0);
col = (col + v1) * v2 * v3;
col = clamp(col, 0.0, 1.0);
return extractAlpha(col);
}
vec4 mainImage(vec2 fragCoord) {
vec2 center = iResolution.xy * 0.5;
float size = min(iResolution.x, iResolution.y);
vec2 uv = (fragCoord - center) / size * 2.0;
float angle = rot;
float s = sin(angle);
float c = cos(angle);
uv = vec2(c * uv.x - s * uv.y, s * uv.x + c * uv.y);
uv.x += hover * hoverIntensity * 0.1 * sin(uv.y * 10.0 + iTime);
uv.y += hover * hoverIntensity * 0.1 * sin(uv.x * 10.0 + iTime);
return draw(uv);
}
void main() {
vec2 fragCoord = vUv * iResolution.xy;
vec4 col = mainImage(fragCoord);
gl_FragColor = vec4(col.rgb * col.a, col.a);
}
`
useEffect(() => {
const container = ctnDom.current
if (!container) return
// Initialize WebGL renderer
const renderer = new Renderer({
alpha: true,
premultipliedAlpha: false,
})
const gl = renderer.gl
gl.clearColor(0, 0, 0, 0)
container.appendChild(gl.canvas)
// Create geometry, program, and mesh
const geometry = new Triangle(gl)
const program = new Program(gl, {
vertex: vert,
fragment: frag,
uniforms: {
iTime: { value: 0 },
iResolution: {
value: new Vec3(
gl.canvas.width,
gl.canvas.height,
gl.canvas.width / gl.canvas.height
),
},
hue: { value: hue },
hover: { value: 0 },
rot: { value: 0 },
hoverIntensity: { value: hoverIntensity },
},
})
const mesh = new Mesh(gl, { geometry, program })
// Handle resize events
function resize() {
if (!container) return
const dpr = window.devicePixelRatio || 1
const width = container.clientWidth
const height = container.clientHeight
renderer.setSize(width * dpr, height * dpr)
gl.canvas.style.width = width + "px"
gl.canvas.style.height = height + "px"
program.uniforms.iResolution.value.set(
gl.canvas.width,
gl.canvas.height,
gl.canvas.width / gl.canvas.height
)
}
// Create resize observer to detect changes in component size on the canvas
const resizeObserver = new ResizeObserver(() => {
resize()
})
// Observe the container for size changes
resizeObserver.observe(container)
// Also listen for window resize events
window.addEventListener("resize", resize)
// Initial resize
resize()
// Handle mouse interactions
let targetHover = 0
let lastTime = 0
let currentRot = 0
const rotationSpeed = 0.3 // radians per second
const handleMouseMove = (e) => {
const rect = container.getBoundingClientRect()
const x = e.clientX - rect.left
const y = e.clientY - rect.top
const width = rect.width
const height = rect.height
const size = Math.min(width, height)
const centerX = width / 2
const centerY = height / 2
const uvX = ((x - centerX) / size) * 2.0
const uvY = ((y - centerY) / size) * 2.0
if (Math.sqrt(uvX * uvX + uvY * uvY) < 0.8) {
targetHover = 1
} else {
targetHover = 0
}
}
const handleMouseLeave = () => {
targetHover = 0
}
// Add event listeners
container.addEventListener("mousemove", handleMouseMove)
container.addEventListener("mouseleave", handleMouseLeave)
// Animation loop
let rafId
const update = (t) => {
rafId = requestAnimationFrame(update)
const dt = (t - lastTime) * 0.001
lastTime = t
program.uniforms.iTime.value = t * 0.001
program.uniforms.hue.value = hue
program.uniforms.hoverIntensity.value = hoverIntensity
const effectiveHover = forceHoverState ? 1 : targetHover
program.uniforms.hover.value +=
(effectiveHover - program.uniforms.hover.value) * 0.1
if (rotateOnHover && effectiveHover > 0.5) {
currentRot += dt * rotationSpeed
}
program.uniforms.rot.value = currentRot
renderer.render({ scene: mesh })
}
rafId = requestAnimationFrame(update)
// Cleanup function
return () => {
cancelAnimationFrame(rafId)
window.removeEventListener("resize", resize)
resizeObserver.disconnect()
container.removeEventListener("mousemove", handleMouseMove)
container.removeEventListener("mouseleave", handleMouseLeave)
container.removeChild(gl.canvas)
gl.getExtension("WEBGL_lose_context")?.loseContext()
}
}, [hue, hoverIntensity, rotateOnHover, forceHoverState, width, height])
return (
<div
ref={ctnDom}
style={{
width: width,
height: height,
position: "relative",
zIndex: 2,
}}
/>
)
}
// Define property controls for Framer
addPropertyControls(Orb, {
hue: {
type: ControlType.Number,
title: "Hue",
min: 0,
max: 360,
step: 1,
defaultValue: 0,
},
hoverIntensity: {
type: ControlType.Number,
title: "Hover Intensity",
min: 0,
max: 1,
step: 0.01,
defaultValue: 0.2,
},
rotateOnHover: {
type: ControlType.Boolean,
title: "Rotate on Hover",
defaultValue: true,
},
forceHoverState: {
type: ControlType.Boolean,
title: "Force Hover",
defaultValue: false,
},
})
CLICK TO COPY
// A custom Framer code component by Chris Kellett - Framerverse
// v1.0.0
import React, { useEffect, useRef } from "react"
import { addPropertyControls, ControlType } from "framer"
// OGL is imported directly in this component
// Mini version of OGL focused on what we need for this component
class Vec3 {
constructor(x = 0, y = 0, z = 0) {
this.x = x
this.y = y
this.z = z
}
set(x, y, z) {
this.x = x
this.y = y
this.z = z
return this
}
}
class Renderer {
constructor(options = {}) {
this.gl = document.createElement("canvas").getContext("webgl", {
alpha: options.alpha || false,
premultipliedAlpha: options.premultipliedAlpha || false,
antialias: options.antialias || false,
})
}
setSize(width, height) {
this.gl.canvas.width = width
this.gl.canvas.height = height
this.gl.viewport(0, 0, width, height)
}
render({ scene }) {
scene.draw()
}
}
class Program {
constructor(gl, { vertex, fragment, uniforms = {} }) {
this.gl = gl
this.uniforms = uniforms
const vertexShader = this.createShader(gl.VERTEX_SHADER, vertex)
const fragmentShader = this.createShader(gl.FRAGMENT_SHADER, fragment)
this.program = gl.createProgram()
gl.attachShader(this.program, vertexShader)
gl.attachShader(this.program, fragmentShader)
gl.linkProgram(this.program)
if (!gl.getProgramParameter(this.program, gl.LINK_STATUS)) {
console.error(
"Failed to link program:",
gl.getProgramInfoLog(this.program)
)
gl.deleteProgram(this.program)
return null
}
// Initialize uniform locations
this.uniformLocations = {}
for (const name in uniforms) {
this.uniformLocations[name] = gl.getUniformLocation(
this.program,
name
)
}
// Initialize attribute locations
this.attributeLocations = {
position: gl.getAttribLocation(this.program, "position"),
uv: gl.getAttribLocation(this.program, "uv"),
}
}
createShader(type, source) {
const gl = this.gl
const shader = gl.createShader(type)
gl.shaderSource(shader, source)
gl.compileShader(shader)
if (!gl.getShaderParameter(shader, gl.COMPILE_STATUS)) {
console.error(
"Failed to compile shader:",
gl.getShaderInfoLog(shader)
)
gl.deleteShader(shader)
return null
}
return shader
}
use() {
this.gl.useProgram(this.program)
this.setUniforms()
return this
}
setUniforms() {
const gl = this.gl
for (const name in this.uniforms) {
const value = this.uniforms[name].value
const location = this.uniformLocations[name]
if (typeof value === "number") {
gl.uniform1f(location, value)
} else if (value instanceof Vec3) {
gl.uniform3f(location, value.x, value.y, value.z)
}
}
}
}
class Triangle {
constructor(gl) {
this.gl = gl
// Define triangle vertices
const vertices = new Float32Array([-1, -1, 3, -1, -1, 3])
// Define UVs
const uvs = new Float32Array([0, 0, 2, 0, 0, 2])
// Create buffers
this.positionBuffer = gl.createBuffer()
gl.bindBuffer(gl.ARRAY_BUFFER, this.positionBuffer)
gl.bufferData(gl.ARRAY_BUFFER, vertices, gl.STATIC_DRAW)
this.uvBuffer = gl.createBuffer()
gl.bindBuffer(gl.ARRAY_BUFFER, this.uvBuffer)
gl.bufferData(gl.ARRAY_BUFFER, uvs, gl.STATIC_DRAW)
}
bind(program) {
const gl = this.gl
gl.bindBuffer(gl.ARRAY_BUFFER, this.positionBuffer)
gl.enableVertexAttribArray(program.attributeLocations.position)
gl.vertexAttribPointer(
program.attributeLocations.position,
2,
gl.FLOAT,
false,
0,
0
)
gl.bindBuffer(gl.ARRAY_BUFFER, this.uvBuffer)
gl.enableVertexAttribArray(program.attributeLocations.uv)
gl.vertexAttribPointer(
program.attributeLocations.uv,
2,
gl.FLOAT,
false,
0,
0
)
}
}
class Mesh {
constructor(gl, { geometry, program }) {
this.gl = gl
this.geometry = geometry
this.program = program
}
draw() {
const gl = this.gl
this.program.use()
this.geometry.bind(this.program)
gl.drawArrays(gl.TRIANGLES, 0, 3)
}
}
// Main Orb component
export default function Orb({
hue = 0,
hoverIntensity = 0.2,
rotateOnHover = true,
forceHoverState = false,
width = "100%",
height = "100%",
}) {
const ctnDom = useRef(null)
// Vertex shader code
const vert = /* glsl */ `
precision highp float;
attribute vec2 position;
attribute vec2 uv;
varying vec2 vUv;
void main() {
vUv = uv;
gl_Position = vec4(position, 0.0, 1.0);
}
`
// Fragment shader code
const frag = /* glsl */ `
precision highp float;
uniform float iTime;
uniform vec3 iResolution;
uniform float hue;
uniform float hover;
uniform float rot;
uniform float hoverIntensity;
varying vec2 vUv;
vec3 rgb2yiq(vec3 c) {
float y = dot(c, vec3(0.299, 0.587, 0.114));
float i = dot(c, vec3(0.596, -0.274, -0.322));
float q = dot(c, vec3(0.211, -0.523, 0.312));
return vec3(y, i, q);
}
vec3 yiq2rgb(vec3 c) {
float r = c.x + 0.956 * c.y + 0.621 * c.z;
float g = c.x - 0.272 * c.y - 0.647 * c.z;
float b = c.x - 1.106 * c.y + 1.703 * c.z;
return vec3(r, g, b);
}
vec3 adjustHue(vec3 color, float hueDeg) {
float hueRad = hueDeg * 3.14159265 / 180.0;
vec3 yiq = rgb2yiq(color);
float cosA = cos(hueRad);
float sinA = sin(hueRad);
float i = yiq.y * cosA - yiq.z * sinA;
float q = yiq.y * sinA + yiq.z * cosA;
yiq.y = i;
yiq.z = q;
return yiq2rgb(yiq);
}
vec3 hash33(vec3 p3) {
p3 = fract(p3 * vec3(0.1031, 0.11369, 0.13787));
p3 += dot(p3, p3.yxz + 19.19);
return -1.0 + 2.0 * fract(vec3(
p3.x + p3.y,
p3.x + p3.z,
p3.y + p3.z
) * p3.zyx);
}
float snoise3(vec3 p) {
const float K1 = 0.333333333;
const float K2 = 0.166666667;
vec3 i = floor(p + (p.x + p.y + p.z) * K1);
vec3 d0 = p - (i - (i.x + i.y + i.z) * K2);
vec3 e = step(vec3(0.0), d0 - d0.yzx);
vec3 i1 = e * (1.0 - e.zxy);
vec3 i2 = 1.0 - e.zxy * (1.0 - e);
vec3 d1 = d0 - (i1 - K2);
vec3 d2 = d0 - (i2 - K1);
vec3 d3 = d0 - 0.5;
vec4 h = max(0.6 - vec4(
dot(d0, d0),
dot(d1, d1),
dot(d2, d2),
dot(d3, d3)
), 0.0);
vec4 n = h * h * h * h * vec4(
dot(d0, hash33(i)),
dot(d1, hash33(i + i1)),
dot(d2, hash33(i + i2)),
dot(d3, hash33(i + 1.0))
);
return dot(vec4(31.316), n);
}
// Instead of "extractAlpha" that normalizes the color,
// we keep the computed color as-is and later multiply by alpha.
vec4 extractAlpha(vec3 colorIn) {
float a = max(max(colorIn.r, colorIn.g), colorIn.b);
return vec4(colorIn.rgb / (a + 1e-5), a);
}
const vec3 baseColor1 = vec3(0.611765, 0.262745, 0.996078);
const vec3 baseColor2 = vec3(0.298039, 0.760784, 0.913725);
const vec3 baseColor3 = vec3(0.062745, 0.078431, 0.600000);
const float innerRadius = 0.6;
const float noiseScale = 0.65;
float light1(float intensity, float attenuation, float dist) {
return intensity / (1.0 + dist * attenuation);
}
float light2(float intensity, float attenuation, float dist) {
return intensity / (1.0 + dist * dist * attenuation);
}
vec4 draw(vec2 uv) {
vec3 color1 = adjustHue(baseColor1, hue);
vec3 color2 = adjustHue(baseColor2, hue);
vec3 color3 = adjustHue(baseColor3, hue);
float ang = atan(uv.y, uv.x);
float len = length(uv);
float invLen = len > 0.0 ? 1.0 / len : 0.0;
float n0 = snoise3(vec3(uv * noiseScale, iTime * 0.5)) * 0.5 + 0.5;
float r0 = mix(mix(innerRadius, 1.0, 0.4), mix(innerRadius, 1.0, 0.6), n0);
float d0 = distance(uv, (r0 * invLen) * uv);
float v0 = light1(1.0, 10.0, d0);
v0 *= smoothstep(r0 * 1.05, r0, len);
float cl = cos(ang + iTime * 2.0) * 0.5 + 0.5;
float a = iTime * -1.0;
vec2 pos = vec2(cos(a), sin(a)) * r0;
float d = distance(uv, pos);
float v1 = light2(1.5, 5.0, d);
v1 *= light1(1.0, 50.0, d0);
float v2 = smoothstep(1.0, mix(innerRadius, 1.0, n0 * 0.5), len);
float v3 = smoothstep(innerRadius, mix(innerRadius, 1.0, 0.5), len);
vec3 col = mix(color1, color2, cl);
col = mix(color3, col, v0);
col = (col + v1) * v2 * v3;
col = clamp(col, 0.0, 1.0);
return extractAlpha(col);
}
vec4 mainImage(vec2 fragCoord) {
vec2 center = iResolution.xy * 0.5;
float size = min(iResolution.x, iResolution.y);
vec2 uv = (fragCoord - center) / size * 2.0;
float angle = rot;
float s = sin(angle);
float c = cos(angle);
uv = vec2(c * uv.x - s * uv.y, s * uv.x + c * uv.y);
uv.x += hover * hoverIntensity * 0.1 * sin(uv.y * 10.0 + iTime);
uv.y += hover * hoverIntensity * 0.1 * sin(uv.x * 10.0 + iTime);
return draw(uv);
}
void main() {
vec2 fragCoord = vUv * iResolution.xy;
vec4 col = mainImage(fragCoord);
gl_FragColor = vec4(col.rgb * col.a, col.a);
}
`
useEffect(() => {
const container = ctnDom.current
if (!container) return
// Initialize WebGL renderer
const renderer = new Renderer({
alpha: true,
premultipliedAlpha: false,
})
const gl = renderer.gl
gl.clearColor(0, 0, 0, 0)
container.appendChild(gl.canvas)
// Create geometry, program, and mesh
const geometry = new Triangle(gl)
const program = new Program(gl, {
vertex: vert,
fragment: frag,
uniforms: {
iTime: { value: 0 },
iResolution: {
value: new Vec3(
gl.canvas.width,
gl.canvas.height,
gl.canvas.width / gl.canvas.height
),
},
hue: { value: hue },
hover: { value: 0 },
rot: { value: 0 },
hoverIntensity: { value: hoverIntensity },
},
})
const mesh = new Mesh(gl, { geometry, program })
// Handle resize events
function resize() {
if (!container) return
const dpr = window.devicePixelRatio || 1
const width = container.clientWidth
const height = container.clientHeight
renderer.setSize(width * dpr, height * dpr)
gl.canvas.style.width = width + "px"
gl.canvas.style.height = height + "px"
program.uniforms.iResolution.value.set(
gl.canvas.width,
gl.canvas.height,
gl.canvas.width / gl.canvas.height
)
}
// Create resize observer to detect changes in component size on the canvas
const resizeObserver = new ResizeObserver(() => {
resize()
})
// Observe the container for size changes
resizeObserver.observe(container)
// Also listen for window resize events
window.addEventListener("resize", resize)
// Initial resize
resize()
// Handle mouse interactions
let targetHover = 0
let lastTime = 0
let currentRot = 0
const rotationSpeed = 0.3 // radians per second
const handleMouseMove = (e) => {
const rect = container.getBoundingClientRect()
const x = e.clientX - rect.left
const y = e.clientY - rect.top
const width = rect.width
const height = rect.height
const size = Math.min(width, height)
const centerX = width / 2
const centerY = height / 2
const uvX = ((x - centerX) / size) * 2.0
const uvY = ((y - centerY) / size) * 2.0
if (Math.sqrt(uvX * uvX + uvY * uvY) < 0.8) {
targetHover = 1
} else {
targetHover = 0
}
}
const handleMouseLeave = () => {
targetHover = 0
}
// Add event listeners
container.addEventListener("mousemove", handleMouseMove)
container.addEventListener("mouseleave", handleMouseLeave)
// Animation loop
let rafId
const update = (t) => {
rafId = requestAnimationFrame(update)
const dt = (t - lastTime) * 0.001
lastTime = t
program.uniforms.iTime.value = t * 0.001
program.uniforms.hue.value = hue
program.uniforms.hoverIntensity.value = hoverIntensity
const effectiveHover = forceHoverState ? 1 : targetHover
program.uniforms.hover.value +=
(effectiveHover - program.uniforms.hover.value) * 0.1
if (rotateOnHover && effectiveHover > 0.5) {
currentRot += dt * rotationSpeed
}
program.uniforms.rot.value = currentRot
renderer.render({ scene: mesh })
}
rafId = requestAnimationFrame(update)
// Cleanup function
return () => {
cancelAnimationFrame(rafId)
window.removeEventListener("resize", resize)
resizeObserver.disconnect()
container.removeEventListener("mousemove", handleMouseMove)
container.removeEventListener("mouseleave", handleMouseLeave)
container.removeChild(gl.canvas)
gl.getExtension("WEBGL_lose_context")?.loseContext()
}
}, [hue, hoverIntensity, rotateOnHover, forceHoverState, width, height])
return (
<div
ref={ctnDom}
style={{
width: width,
height: height,
position: "relative",
zIndex: 2,
}}
/>
)
}
// Define property controls for Framer
addPropertyControls(Orb, {
hue: {
type: ControlType.Number,
title: "Hue",
min: 0,
max: 360,
step: 1,
defaultValue: 0,
},
hoverIntensity: {
type: ControlType.Number,
title: "Hover Intensity",
min: 0,
max: 1,
step: 0.01,
defaultValue: 0.2,
},
rotateOnHover: {
type: ControlType.Boolean,
title: "Rotate on Hover",
defaultValue: true,
},
forceHoverState: {
type: ControlType.Boolean,
title: "Force Hover",
defaultValue: false,
},
})
CLICK TO COPY
// A custom Framer code component by Chris Kellett - Framerverse
// v1.0.0
import React, { useEffect, useRef } from "react"
import { addPropertyControls, ControlType } from "framer"
// OGL is imported directly in this component
// Mini version of OGL focused on what we need for this component
class Vec3 {
constructor(x = 0, y = 0, z = 0) {
this.x = x
this.y = y
this.z = z
}
set(x, y, z) {
this.x = x
this.y = y
this.z = z
return this
}
}
class Renderer {
constructor(options = {}) {
this.gl = document.createElement("canvas").getContext("webgl", {
alpha: options.alpha || false,
premultipliedAlpha: options.premultipliedAlpha || false,
antialias: options.antialias || false,
})
}
setSize(width, height) {
this.gl.canvas.width = width
this.gl.canvas.height = height
this.gl.viewport(0, 0, width, height)
}
render({ scene }) {
scene.draw()
}
}
class Program {
constructor(gl, { vertex, fragment, uniforms = {} }) {
this.gl = gl
this.uniforms = uniforms
const vertexShader = this.createShader(gl.VERTEX_SHADER, vertex)
const fragmentShader = this.createShader(gl.FRAGMENT_SHADER, fragment)
this.program = gl.createProgram()
gl.attachShader(this.program, vertexShader)
gl.attachShader(this.program, fragmentShader)
gl.linkProgram(this.program)
if (!gl.getProgramParameter(this.program, gl.LINK_STATUS)) {
console.error(
"Failed to link program:",
gl.getProgramInfoLog(this.program)
)
gl.deleteProgram(this.program)
return null
}
// Initialize uniform locations
this.uniformLocations = {}
for (const name in uniforms) {
this.uniformLocations[name] = gl.getUniformLocation(
this.program,
name
)
}
// Initialize attribute locations
this.attributeLocations = {
position: gl.getAttribLocation(this.program, "position"),
uv: gl.getAttribLocation(this.program, "uv"),
}
}
createShader(type, source) {
const gl = this.gl
const shader = gl.createShader(type)
gl.shaderSource(shader, source)
gl.compileShader(shader)
if (!gl.getShaderParameter(shader, gl.COMPILE_STATUS)) {
console.error(
"Failed to compile shader:",
gl.getShaderInfoLog(shader)
)
gl.deleteShader(shader)
return null
}
return shader
}
use() {
this.gl.useProgram(this.program)
this.setUniforms()
return this
}
setUniforms() {
const gl = this.gl
for (const name in this.uniforms) {
const value = this.uniforms[name].value
const location = this.uniformLocations[name]
if (typeof value === "number") {
gl.uniform1f(location, value)
} else if (value instanceof Vec3) {
gl.uniform3f(location, value.x, value.y, value.z)
}
}
}
}
class Triangle {
constructor(gl) {
this.gl = gl
// Define triangle vertices
const vertices = new Float32Array([-1, -1, 3, -1, -1, 3])
// Define UVs
const uvs = new Float32Array([0, 0, 2, 0, 0, 2])
// Create buffers
this.positionBuffer = gl.createBuffer()
gl.bindBuffer(gl.ARRAY_BUFFER, this.positionBuffer)
gl.bufferData(gl.ARRAY_BUFFER, vertices, gl.STATIC_DRAW)
this.uvBuffer = gl.createBuffer()
gl.bindBuffer(gl.ARRAY_BUFFER, this.uvBuffer)
gl.bufferData(gl.ARRAY_BUFFER, uvs, gl.STATIC_DRAW)
}
bind(program) {
const gl = this.gl
gl.bindBuffer(gl.ARRAY_BUFFER, this.positionBuffer)
gl.enableVertexAttribArray(program.attributeLocations.position)
gl.vertexAttribPointer(
program.attributeLocations.position,
2,
gl.FLOAT,
false,
0,
0
)
gl.bindBuffer(gl.ARRAY_BUFFER, this.uvBuffer)
gl.enableVertexAttribArray(program.attributeLocations.uv)
gl.vertexAttribPointer(
program.attributeLocations.uv,
2,
gl.FLOAT,
false,
0,
0
)
}
}
class Mesh {
constructor(gl, { geometry, program }) {
this.gl = gl
this.geometry = geometry
this.program = program
}
draw() {
const gl = this.gl
this.program.use()
this.geometry.bind(this.program)
gl.drawArrays(gl.TRIANGLES, 0, 3)
}
}
// Main Orb component
export default function Orb({
hue = 0,
hoverIntensity = 0.2,
rotateOnHover = true,
forceHoverState = false,
width = "100%",
height = "100%",
}) {
const ctnDom = useRef(null)
// Vertex shader code
const vert = /* glsl */ `
precision highp float;
attribute vec2 position;
attribute vec2 uv;
varying vec2 vUv;
void main() {
vUv = uv;
gl_Position = vec4(position, 0.0, 1.0);
}
`
// Fragment shader code
const frag = /* glsl */ `
precision highp float;
uniform float iTime;
uniform vec3 iResolution;
uniform float hue;
uniform float hover;
uniform float rot;
uniform float hoverIntensity;
varying vec2 vUv;
vec3 rgb2yiq(vec3 c) {
float y = dot(c, vec3(0.299, 0.587, 0.114));
float i = dot(c, vec3(0.596, -0.274, -0.322));
float q = dot(c, vec3(0.211, -0.523, 0.312));
return vec3(y, i, q);
}
vec3 yiq2rgb(vec3 c) {
float r = c.x + 0.956 * c.y + 0.621 * c.z;
float g = c.x - 0.272 * c.y - 0.647 * c.z;
float b = c.x - 1.106 * c.y + 1.703 * c.z;
return vec3(r, g, b);
}
vec3 adjustHue(vec3 color, float hueDeg) {
float hueRad = hueDeg * 3.14159265 / 180.0;
vec3 yiq = rgb2yiq(color);
float cosA = cos(hueRad);
float sinA = sin(hueRad);
float i = yiq.y * cosA - yiq.z * sinA;
float q = yiq.y * sinA + yiq.z * cosA;
yiq.y = i;
yiq.z = q;
return yiq2rgb(yiq);
}
vec3 hash33(vec3 p3) {
p3 = fract(p3 * vec3(0.1031, 0.11369, 0.13787));
p3 += dot(p3, p3.yxz + 19.19);
return -1.0 + 2.0 * fract(vec3(
p3.x + p3.y,
p3.x + p3.z,
p3.y + p3.z
) * p3.zyx);
}
float snoise3(vec3 p) {
const float K1 = 0.333333333;
const float K2 = 0.166666667;
vec3 i = floor(p + (p.x + p.y + p.z) * K1);
vec3 d0 = p - (i - (i.x + i.y + i.z) * K2);
vec3 e = step(vec3(0.0), d0 - d0.yzx);
vec3 i1 = e * (1.0 - e.zxy);
vec3 i2 = 1.0 - e.zxy * (1.0 - e);
vec3 d1 = d0 - (i1 - K2);
vec3 d2 = d0 - (i2 - K1);
vec3 d3 = d0 - 0.5;
vec4 h = max(0.6 - vec4(
dot(d0, d0),
dot(d1, d1),
dot(d2, d2),
dot(d3, d3)
), 0.0);
vec4 n = h * h * h * h * vec4(
dot(d0, hash33(i)),
dot(d1, hash33(i + i1)),
dot(d2, hash33(i + i2)),
dot(d3, hash33(i + 1.0))
);
return dot(vec4(31.316), n);
}
// Instead of "extractAlpha" that normalizes the color,
// we keep the computed color as-is and later multiply by alpha.
vec4 extractAlpha(vec3 colorIn) {
float a = max(max(colorIn.r, colorIn.g), colorIn.b);
return vec4(colorIn.rgb / (a + 1e-5), a);
}
const vec3 baseColor1 = vec3(0.611765, 0.262745, 0.996078);
const vec3 baseColor2 = vec3(0.298039, 0.760784, 0.913725);
const vec3 baseColor3 = vec3(0.062745, 0.078431, 0.600000);
const float innerRadius = 0.6;
const float noiseScale = 0.65;
float light1(float intensity, float attenuation, float dist) {
return intensity / (1.0 + dist * attenuation);
}
float light2(float intensity, float attenuation, float dist) {
return intensity / (1.0 + dist * dist * attenuation);
}
vec4 draw(vec2 uv) {
vec3 color1 = adjustHue(baseColor1, hue);
vec3 color2 = adjustHue(baseColor2, hue);
vec3 color3 = adjustHue(baseColor3, hue);
float ang = atan(uv.y, uv.x);
float len = length(uv);
float invLen = len > 0.0 ? 1.0 / len : 0.0;
float n0 = snoise3(vec3(uv * noiseScale, iTime * 0.5)) * 0.5 + 0.5;
float r0 = mix(mix(innerRadius, 1.0, 0.4), mix(innerRadius, 1.0, 0.6), n0);
float d0 = distance(uv, (r0 * invLen) * uv);
float v0 = light1(1.0, 10.0, d0);
v0 *= smoothstep(r0 * 1.05, r0, len);
float cl = cos(ang + iTime * 2.0) * 0.5 + 0.5;
float a = iTime * -1.0;
vec2 pos = vec2(cos(a), sin(a)) * r0;
float d = distance(uv, pos);
float v1 = light2(1.5, 5.0, d);
v1 *= light1(1.0, 50.0, d0);
float v2 = smoothstep(1.0, mix(innerRadius, 1.0, n0 * 0.5), len);
float v3 = smoothstep(innerRadius, mix(innerRadius, 1.0, 0.5), len);
vec3 col = mix(color1, color2, cl);
col = mix(color3, col, v0);
col = (col + v1) * v2 * v3;
col = clamp(col, 0.0, 1.0);
return extractAlpha(col);
}
vec4 mainImage(vec2 fragCoord) {
vec2 center = iResolution.xy * 0.5;
float size = min(iResolution.x, iResolution.y);
vec2 uv = (fragCoord - center) / size * 2.0;
float angle = rot;
float s = sin(angle);
float c = cos(angle);
uv = vec2(c * uv.x - s * uv.y, s * uv.x + c * uv.y);
uv.x += hover * hoverIntensity * 0.1 * sin(uv.y * 10.0 + iTime);
uv.y += hover * hoverIntensity * 0.1 * sin(uv.x * 10.0 + iTime);
return draw(uv);
}
void main() {
vec2 fragCoord = vUv * iResolution.xy;
vec4 col = mainImage(fragCoord);
gl_FragColor = vec4(col.rgb * col.a, col.a);
}
`
useEffect(() => {
const container = ctnDom.current
if (!container) return
// Initialize WebGL renderer
const renderer = new Renderer({
alpha: true,
premultipliedAlpha: false,
})
const gl = renderer.gl
gl.clearColor(0, 0, 0, 0)
container.appendChild(gl.canvas)
// Create geometry, program, and mesh
const geometry = new Triangle(gl)
const program = new Program(gl, {
vertex: vert,
fragment: frag,
uniforms: {
iTime: { value: 0 },
iResolution: {
value: new Vec3(
gl.canvas.width,
gl.canvas.height,
gl.canvas.width / gl.canvas.height
),
},
hue: { value: hue },
hover: { value: 0 },
rot: { value: 0 },
hoverIntensity: { value: hoverIntensity },
},
})
const mesh = new Mesh(gl, { geometry, program })
// Handle resize events
function resize() {
if (!container) return
const dpr = window.devicePixelRatio || 1
const width = container.clientWidth
const height = container.clientHeight
renderer.setSize(width * dpr, height * dpr)
gl.canvas.style.width = width + "px"
gl.canvas.style.height = height + "px"
program.uniforms.iResolution.value.set(
gl.canvas.width,
gl.canvas.height,
gl.canvas.width / gl.canvas.height
)
}
// Create resize observer to detect changes in component size on the canvas
const resizeObserver = new ResizeObserver(() => {
resize()
})
// Observe the container for size changes
resizeObserver.observe(container)
// Also listen for window resize events
window.addEventListener("resize", resize)
// Initial resize
resize()
// Handle mouse interactions
let targetHover = 0
let lastTime = 0
let currentRot = 0
const rotationSpeed = 0.3 // radians per second
const handleMouseMove = (e) => {
const rect = container.getBoundingClientRect()
const x = e.clientX - rect.left
const y = e.clientY - rect.top
const width = rect.width
const height = rect.height
const size = Math.min(width, height)
const centerX = width / 2
const centerY = height / 2
const uvX = ((x - centerX) / size) * 2.0
const uvY = ((y - centerY) / size) * 2.0
if (Math.sqrt(uvX * uvX + uvY * uvY) < 0.8) {
targetHover = 1
} else {
targetHover = 0
}
}
const handleMouseLeave = () => {
targetHover = 0
}
// Add event listeners
container.addEventListener("mousemove", handleMouseMove)
container.addEventListener("mouseleave", handleMouseLeave)
// Animation loop
let rafId
const update = (t) => {
rafId = requestAnimationFrame(update)
const dt = (t - lastTime) * 0.001
lastTime = t
program.uniforms.iTime.value = t * 0.001
program.uniforms.hue.value = hue
program.uniforms.hoverIntensity.value = hoverIntensity
const effectiveHover = forceHoverState ? 1 : targetHover
program.uniforms.hover.value +=
(effectiveHover - program.uniforms.hover.value) * 0.1
if (rotateOnHover && effectiveHover > 0.5) {
currentRot += dt * rotationSpeed
}
program.uniforms.rot.value = currentRot
renderer.render({ scene: mesh })
}
rafId = requestAnimationFrame(update)
// Cleanup function
return () => {
cancelAnimationFrame(rafId)
window.removeEventListener("resize", resize)
resizeObserver.disconnect()
container.removeEventListener("mousemove", handleMouseMove)
container.removeEventListener("mouseleave", handleMouseLeave)
container.removeChild(gl.canvas)
gl.getExtension("WEBGL_lose_context")?.loseContext()
}
}, [hue, hoverIntensity, rotateOnHover, forceHoverState, width, height])
return (
<div
ref={ctnDom}
style={{
width: width,
height: height,
position: "relative",
zIndex: 2,
}}
/>
)
}
// Define property controls for Framer
addPropertyControls(Orb, {
hue: {
type: ControlType.Number,
title: "Hue",
min: 0,
max: 360,
step: 1,
defaultValue: 0,
},
hoverIntensity: {
type: ControlType.Number,
title: "Hover Intensity",
min: 0,
max: 1,
step: 0.01,
defaultValue: 0.2,
},
rotateOnHover: {
type: ControlType.Boolean,
title: "Rotate on Hover",
defaultValue: true,
},
forceHoverState: {
type: ControlType.Boolean,
title: "Force Hover",
defaultValue: false,
},
})
CLICK TO COPY
What is it?
A very cool background effect great for startups.
What is it?
A very cool background effect great for startups.
What is it?
A very cool background effect great for startups.
How to use.
Click in the code are below to copy the code to your clipboard. Then click the 'plus' button int the assets panel for 'Code'. Choose if its an overide or Component. Paste the code in and save.
How to use.
Click in the code are below to copy the code to your clipboard. Then click the 'plus' button int the assets panel for 'Code'. Choose if its an overide or Component. Paste the code in and save.
How to use.
Click in the code are below to copy the code to your clipboard. Then click the 'plus' button int the assets panel for 'Code'. Choose if its an overide or Component. Paste the code in and save.
Usage Licence
I'm happy for you to use this code in your projects, even templates. If you use it please keep my accreditation in the code. Please don't sell it as your own. (Hover for full licence).
Usage Licence
I'm happy for you to use this code in your projects, even templates. If you use it please keep my accreditation in the code. Please don't sell it as your own. (Hover for full licence).
Usage Licence
I'm happy for you to use this code in your projects, even templates. If you use it please keep my accreditation in the code. Please don't sell it as your own. (Hover for full licence).
Change Log
//Version 1
Figma to Framer
Support
If you need support first watch the help video above. If that does not help reach out to me on one of my social channels or use the contact form. As I am a team of one it may take a short while to get back to you. Please be patient, thanks.
Hope this helps. Good luck with your project.
Scan to open on mobile.
More Framer resources.

CMS Drivend Password‑protected Client Pages
Secure any collection page with a drag‑and‑drop lockout that remembers each visitor — no code, no servers, just Framer.
Component

CMS Drivend Password‑protected Client Pages
Secure any collection page with a drag‑and‑drop lockout that remembers each visitor — no code, no servers, just Framer.
Component

CMS Drivend Password‑protected Client Pages
Secure any collection page with a drag‑and‑drop lockout that remembers each visitor — no code, no servers, just Framer.
Component

Dynamic QR Maker
Easily add auto generated QR codes to anything Framer
Code Component

Dynamic QR Maker
Easily add auto generated QR codes to anything Framer
Code Component

Dynamic QR Maker
Easily add auto generated QR codes to anything Framer
Code Component

Popup Advert Kit for Framer
Everything you need to start adding and running professional popup ads on Framer sites.
Kit

Popup Advert Kit for Framer
Everything you need to start adding and running professional popup ads on Framer sites.
Kit

Popup Advert Kit for Framer
Everything you need to start adding and running professional popup ads on Framer sites.
Kit
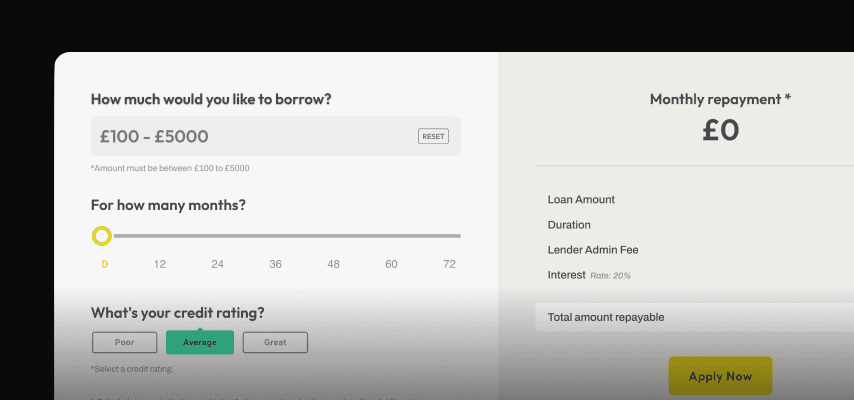
Real Loan Calculator
Add a fully functional, responsive, configurable loan calculator to your project.
Section
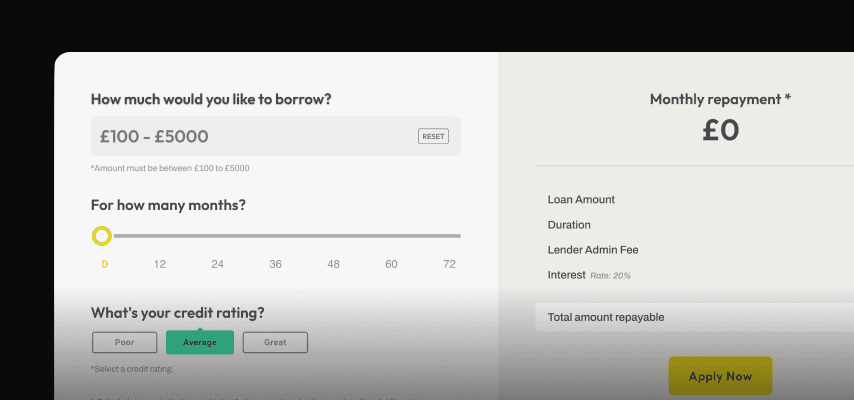
Real Loan Calculator
Add a fully functional, responsive, configurable loan calculator to your project.
Section
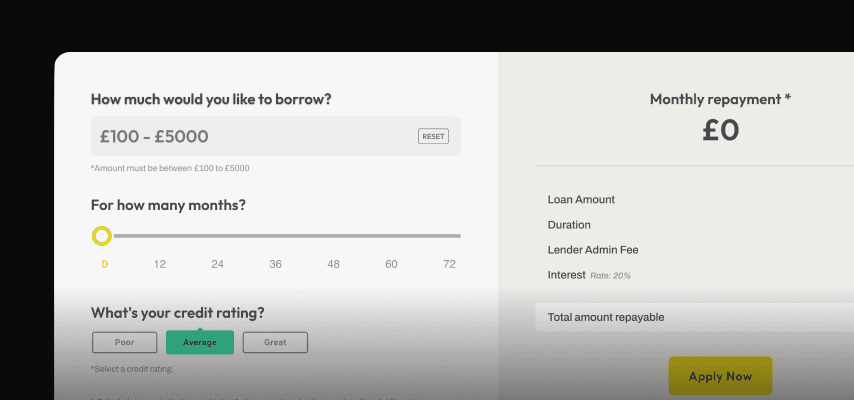
Real Loan Calculator
Add a fully functional, responsive, configurable loan calculator to your project.
Section
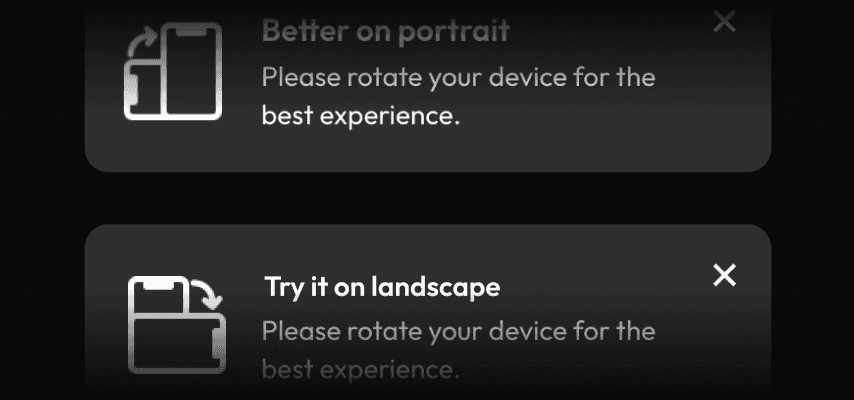
Mobile Rotation Alert
If your site works better in one orientation than another why not let your users know with this little component.
Code Component
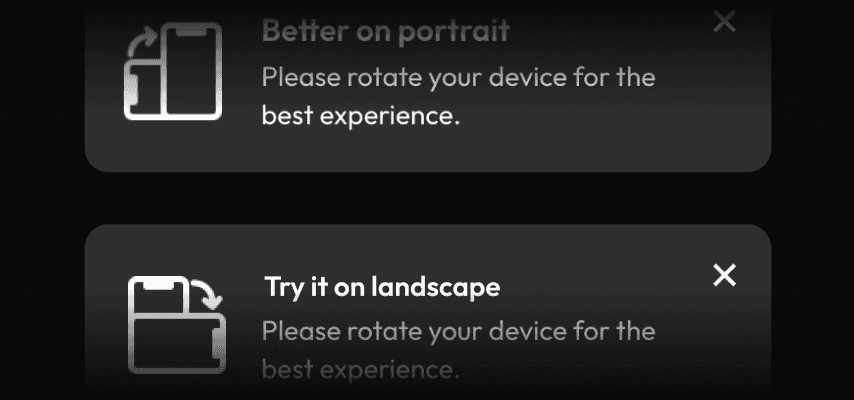
Mobile Rotation Alert
If your site works better in one orientation than another why not let your users know with this little component.
Code Component
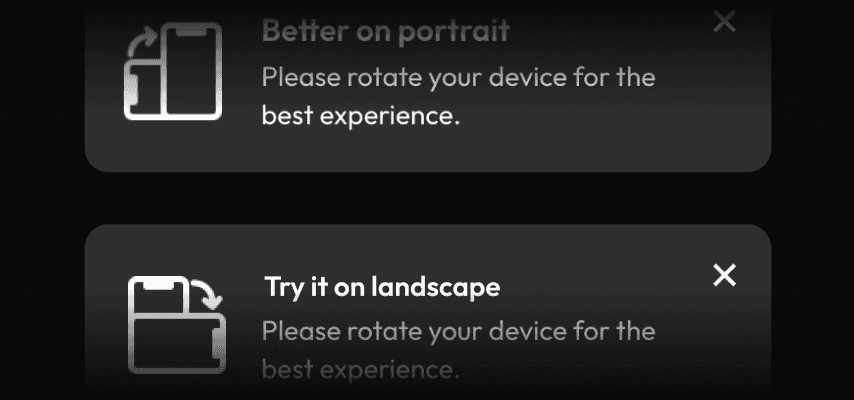
Mobile Rotation Alert
If your site works better in one orientation than another why not let your users know with this little component.
Code Component
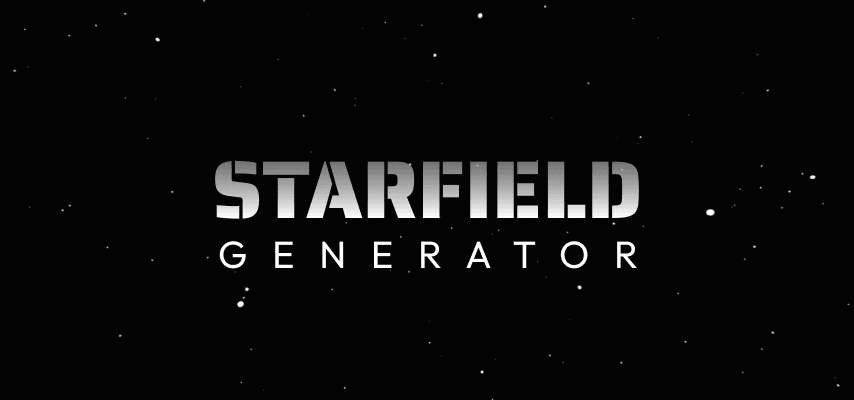
Starfield Generator
A niche component for creating a swirling Starfield, simple but great in the right scenario (like this site).
Code Component
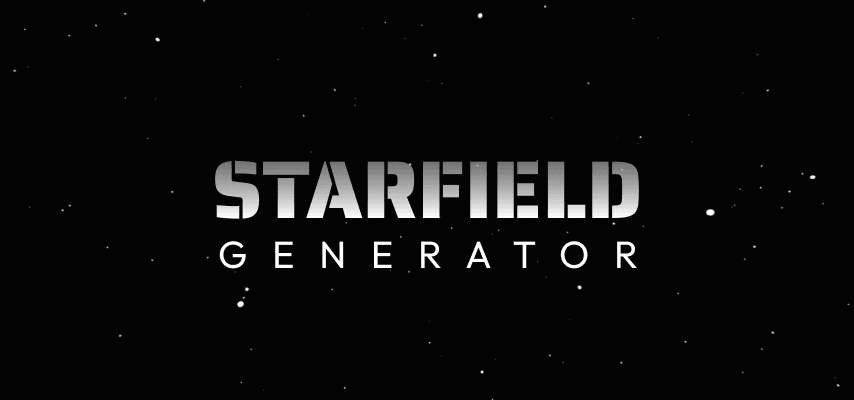
Starfield Generator
A niche component for creating a swirling Starfield, simple but great in the right scenario (like this site).
Code Component
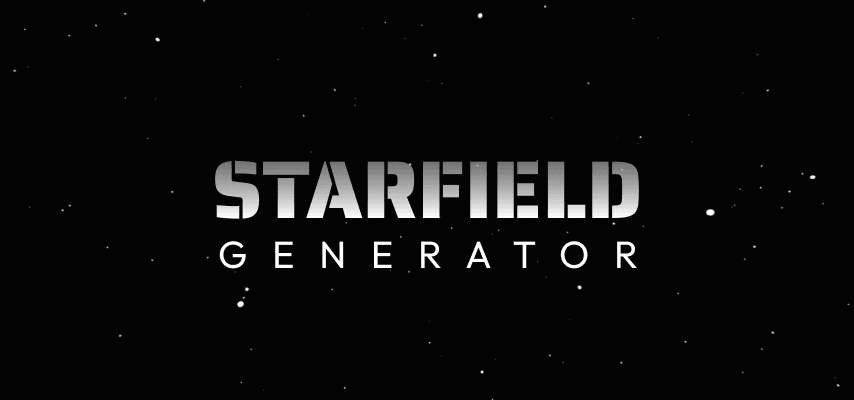
Starfield Generator
A niche component for creating a swirling Starfield, simple but great in the right scenario (like this site).
Code Component