Back
Back
Back
Starfield Generator
Starfield Generator
Page Contents
Page Contents
Page Contents
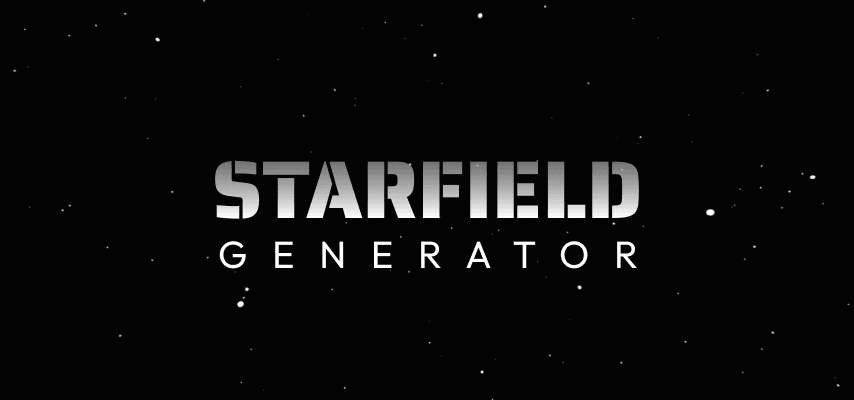
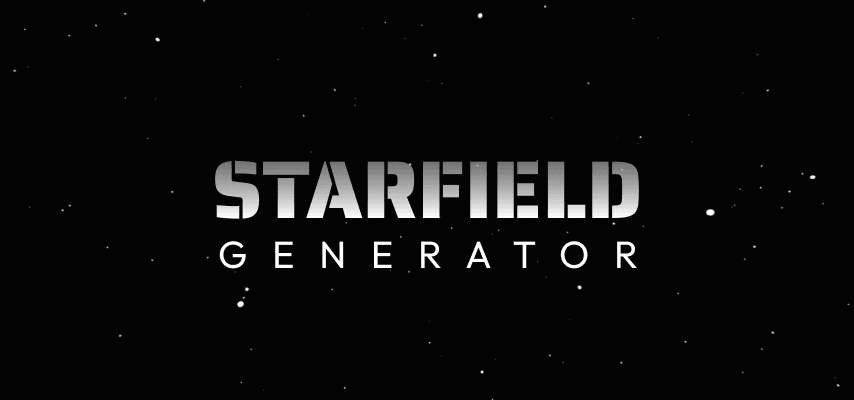
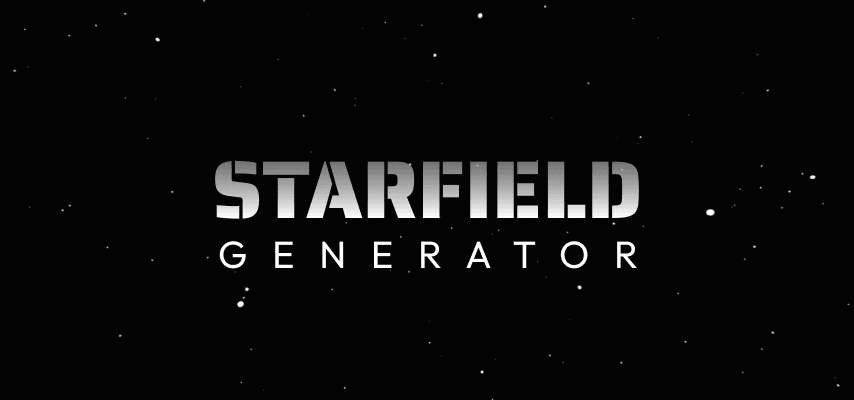
A niche component for creating a swirling Starfield, simple but great in the right scenario (like this site).
When I first started building Framerverse, I envisioned a swirling starfield background, and over time, that idea evolved into this component.
It’s built using Fiber 3D for React and took quite a bit of tweaking to get just right. It includes a single parameter for controlling the speed of the stars, and I love how it turned out. I wanted to share it with you.
I’m not sure when you might need it, but if you do, I hope it’s exactly what you’re looking for. As always, you’re welcome!
If you are not sure how to add code to Framer watch this quick video.
Framer Code Component
Starfield Generator
1.0
1.0
1.0
1.0
// A custom Framer code override by Chris Kellett - Framerverse
// Get more components at www.framerverse.com
// Starfield Generator
// Version 1.0
import React, { useEffect, useRef } from "react"
import { motion } from "framer-motion"
import * as THREE from "three"
import { addPropertyControls, ControlType } from "framer"
function StarFieldGenerator({ speed }) {
return (
<motion.div
style={{
width: "100%",
height: "100%",
display: "flex",
justifyContent: "center",
alignItems: "center",
background: "transparent",
}}
>
<StarfieldAnimation speed={speed} />
</motion.div>
)
}
function StarfieldAnimation({ speed }) {
const mountRef = useRef(null)
useEffect(() => {
const mount = mountRef.current
let width = mount.clientWidth
let height = mount.clientHeight
// Set up scene
const scene = new THREE.Scene()
// Set up camera
const camera = new THREE.PerspectiveCamera(
75,
width / height,
0.1,
1000
)
camera.position.set(0, 0, 0)
// Set up renderer
const renderer = new THREE.WebGLRenderer({
alpha: true,
antialias: true,
})
renderer.setSize(width, height)
renderer.setClearColor(0x000000, 0) // Set background to transparent
mount.appendChild(renderer.domElement)
// Array to store stars
const stars = []
// Function to create a star
function createStar() {
const starGeometry = new THREE.SphereGeometry(
Math.random() * 2 + 0.5,
32,
32
)
const starMaterial = new THREE.MeshBasicMaterial({
color: 0xffffff,
})
const star = new THREE.Mesh(starGeometry, starMaterial)
const distance = Math.random() * 1000 + 500
const angle = Math.random() * Math.PI * 2
star.position.set(
Math.cos(angle) * distance,
Math.sin(angle) * distance,
-Math.random() * 500
)
scene.add(star)
stars.push(star)
}
// Create stars
const numStars = 500
for (let i = 0; i < numStars; i++) {
createStar()
}
// Set up animation loop
const animate = () => {
requestAnimationFrame(animate)
// Move stars towards the camera and update positions
stars.forEach((star) => {
star.position.z += speed
if (star.position.z > 0) {
star.position.z = -1000
star.position.x = (Math.random() - 0.5) * 2000
star.position.y = (Math.random() - 0.5) * 2000
}
})
// Rotate camera for barrel roll effect
camera.rotation.z += 0.001
renderer.render(scene, camera)
}
animate()
// Use ResizeObserver to handle resize
const resizeObserver = new ResizeObserver(() => {
width = mount.clientWidth
height = mount.clientHeight
camera.aspect = width / height
camera.updateProjectionMatrix()
renderer.setSize(width, height)
})
resizeObserver.observe(mount)
return () => {
resizeObserver.disconnect()
if (mount && renderer.domElement) {
mount.removeChild(renderer.domElement)
}
}
}, [speed])
return <div ref={mountRef} style={{ width: "100%", height: "100%" }} />
}
// Add property controls for Framer
addPropertyControls(StarFieldGenerator, {
speed: {
type: ControlType.Number,
title: "Speed",
defaultValue: 1,
min: 0.1,
max: 10,
step: 0.1,
},
})
export { StarFieldGenerator as default }
CLICK TO COPY
// A custom Framer code override by Chris Kellett - Framerverse
// Get more components at www.framerverse.com
// Starfield Generator
// Version 1.0
import React, { useEffect, useRef } from "react"
import { motion } from "framer-motion"
import * as THREE from "three"
import { addPropertyControls, ControlType } from "framer"
function StarFieldGenerator({ speed }) {
return (
<motion.div
style={{
width: "100%",
height: "100%",
display: "flex",
justifyContent: "center",
alignItems: "center",
background: "transparent",
}}
>
<StarfieldAnimation speed={speed} />
</motion.div>
)
}
function StarfieldAnimation({ speed }) {
const mountRef = useRef(null)
useEffect(() => {
const mount = mountRef.current
let width = mount.clientWidth
let height = mount.clientHeight
// Set up scene
const scene = new THREE.Scene()
// Set up camera
const camera = new THREE.PerspectiveCamera(
75,
width / height,
0.1,
1000
)
camera.position.set(0, 0, 0)
// Set up renderer
const renderer = new THREE.WebGLRenderer({
alpha: true,
antialias: true,
})
renderer.setSize(width, height)
renderer.setClearColor(0x000000, 0) // Set background to transparent
mount.appendChild(renderer.domElement)
// Array to store stars
const stars = []
// Function to create a star
function createStar() {
const starGeometry = new THREE.SphereGeometry(
Math.random() * 2 + 0.5,
32,
32
)
const starMaterial = new THREE.MeshBasicMaterial({
color: 0xffffff,
})
const star = new THREE.Mesh(starGeometry, starMaterial)
const distance = Math.random() * 1000 + 500
const angle = Math.random() * Math.PI * 2
star.position.set(
Math.cos(angle) * distance,
Math.sin(angle) * distance,
-Math.random() * 500
)
scene.add(star)
stars.push(star)
}
// Create stars
const numStars = 500
for (let i = 0; i < numStars; i++) {
createStar()
}
// Set up animation loop
const animate = () => {
requestAnimationFrame(animate)
// Move stars towards the camera and update positions
stars.forEach((star) => {
star.position.z += speed
if (star.position.z > 0) {
star.position.z = -1000
star.position.x = (Math.random() - 0.5) * 2000
star.position.y = (Math.random() - 0.5) * 2000
}
})
// Rotate camera for barrel roll effect
camera.rotation.z += 0.001
renderer.render(scene, camera)
}
animate()
// Use ResizeObserver to handle resize
const resizeObserver = new ResizeObserver(() => {
width = mount.clientWidth
height = mount.clientHeight
camera.aspect = width / height
camera.updateProjectionMatrix()
renderer.setSize(width, height)
})
resizeObserver.observe(mount)
return () => {
resizeObserver.disconnect()
if (mount && renderer.domElement) {
mount.removeChild(renderer.domElement)
}
}
}, [speed])
return <div ref={mountRef} style={{ width: "100%", height: "100%" }} />
}
// Add property controls for Framer
addPropertyControls(StarFieldGenerator, {
speed: {
type: ControlType.Number,
title: "Speed",
defaultValue: 1,
min: 0.1,
max: 10,
step: 0.1,
},
})
export { StarFieldGenerator as default }
CLICK TO COPY
// A custom Framer code override by Chris Kellett - Framerverse
// Get more components at www.framerverse.com
// Starfield Generator
// Version 1.0
import React, { useEffect, useRef } from "react"
import { motion } from "framer-motion"
import * as THREE from "three"
import { addPropertyControls, ControlType } from "framer"
function StarFieldGenerator({ speed }) {
return (
<motion.div
style={{
width: "100%",
height: "100%",
display: "flex",
justifyContent: "center",
alignItems: "center",
background: "transparent",
}}
>
<StarfieldAnimation speed={speed} />
</motion.div>
)
}
function StarfieldAnimation({ speed }) {
const mountRef = useRef(null)
useEffect(() => {
const mount = mountRef.current
let width = mount.clientWidth
let height = mount.clientHeight
// Set up scene
const scene = new THREE.Scene()
// Set up camera
const camera = new THREE.PerspectiveCamera(
75,
width / height,
0.1,
1000
)
camera.position.set(0, 0, 0)
// Set up renderer
const renderer = new THREE.WebGLRenderer({
alpha: true,
antialias: true,
})
renderer.setSize(width, height)
renderer.setClearColor(0x000000, 0) // Set background to transparent
mount.appendChild(renderer.domElement)
// Array to store stars
const stars = []
// Function to create a star
function createStar() {
const starGeometry = new THREE.SphereGeometry(
Math.random() * 2 + 0.5,
32,
32
)
const starMaterial = new THREE.MeshBasicMaterial({
color: 0xffffff,
})
const star = new THREE.Mesh(starGeometry, starMaterial)
const distance = Math.random() * 1000 + 500
const angle = Math.random() * Math.PI * 2
star.position.set(
Math.cos(angle) * distance,
Math.sin(angle) * distance,
-Math.random() * 500
)
scene.add(star)
stars.push(star)
}
// Create stars
const numStars = 500
for (let i = 0; i < numStars; i++) {
createStar()
}
// Set up animation loop
const animate = () => {
requestAnimationFrame(animate)
// Move stars towards the camera and update positions
stars.forEach((star) => {
star.position.z += speed
if (star.position.z > 0) {
star.position.z = -1000
star.position.x = (Math.random() - 0.5) * 2000
star.position.y = (Math.random() - 0.5) * 2000
}
})
// Rotate camera for barrel roll effect
camera.rotation.z += 0.001
renderer.render(scene, camera)
}
animate()
// Use ResizeObserver to handle resize
const resizeObserver = new ResizeObserver(() => {
width = mount.clientWidth
height = mount.clientHeight
camera.aspect = width / height
camera.updateProjectionMatrix()
renderer.setSize(width, height)
})
resizeObserver.observe(mount)
return () => {
resizeObserver.disconnect()
if (mount && renderer.domElement) {
mount.removeChild(renderer.domElement)
}
}
}, [speed])
return <div ref={mountRef} style={{ width: "100%", height: "100%" }} />
}
// Add property controls for Framer
addPropertyControls(StarFieldGenerator, {
speed: {
type: ControlType.Number,
title: "Speed",
defaultValue: 1,
min: 0.1,
max: 10,
step: 0.1,
},
})
export { StarFieldGenerator as default }
CLICK TO COPY
What is it?
A niche component for creating a swirling Starfield, simple but great in the right scenario (like this site).
What is it?
A niche component for creating a swirling Starfield, simple but great in the right scenario (like this site).
What is it?
A niche component for creating a swirling Starfield, simple but great in the right scenario (like this site).
How to use.
Click in the code are below to copy the code to your clipboard. Then click the 'plus' button int the assets panel for 'Code'. Choose if its an overide or Component. Paste the code in and save.
How to use.
Click in the code are below to copy the code to your clipboard. Then click the 'plus' button int the assets panel for 'Code'. Choose if its an overide or Component. Paste the code in and save.
How to use.
Click in the code are below to copy the code to your clipboard. Then click the 'plus' button int the assets panel for 'Code'. Choose if its an overide or Component. Paste the code in and save.
Usage Licence
I'm happy for you to use this code in your projects, even templates. If you use it please keep my accreditation in the code. Please don't sell it as your own. (Hover for full licence).
Usage Licence
I'm happy for you to use this code in your projects, even templates. If you use it please keep my accreditation in the code. Please don't sell it as your own. (Hover for full licence).
Usage Licence
I'm happy for you to use this code in your projects, even templates. If you use it please keep my accreditation in the code. Please don't sell it as your own. (Hover for full licence).
Change Log
Figma to Framer
Support
If you need support first watch the help video above. If that does not help reach out to me on one of my social channels or use the contact form. As I am a team of one it may take a short while to get back to you. Please be patient, thanks.
Hope this helps. Good luck with your project.
Scan to open on mobile.
More Framer resources.

CMS Drivend Password‑protected Client Pages
Secure any collection page with a drag‑and‑drop lockout that remembers each visitor — no code, no servers, just Framer.
Component

CMS Drivend Password‑protected Client Pages
Secure any collection page with a drag‑and‑drop lockout that remembers each visitor — no code, no servers, just Framer.
Component

CMS Drivend Password‑protected Client Pages
Secure any collection page with a drag‑and‑drop lockout that remembers each visitor — no code, no servers, just Framer.
Component

Dynamic QR Maker
Easily add auto generated QR codes to anything Framer
Code Component

Dynamic QR Maker
Easily add auto generated QR codes to anything Framer
Code Component

Dynamic QR Maker
Easily add auto generated QR codes to anything Framer
Code Component

Orb Background
A very cool background effect great for startups.
Code Component

Orb Background
A very cool background effect great for startups.
Code Component

Orb Background
A very cool background effect great for startups.
Code Component

Popup Advert Kit for Framer
Everything you need to start adding and running professional popup ads on Framer sites.
Kit

Popup Advert Kit for Framer
Everything you need to start adding and running professional popup ads on Framer sites.
Kit

Popup Advert Kit for Framer
Everything you need to start adding and running professional popup ads on Framer sites.
Kit
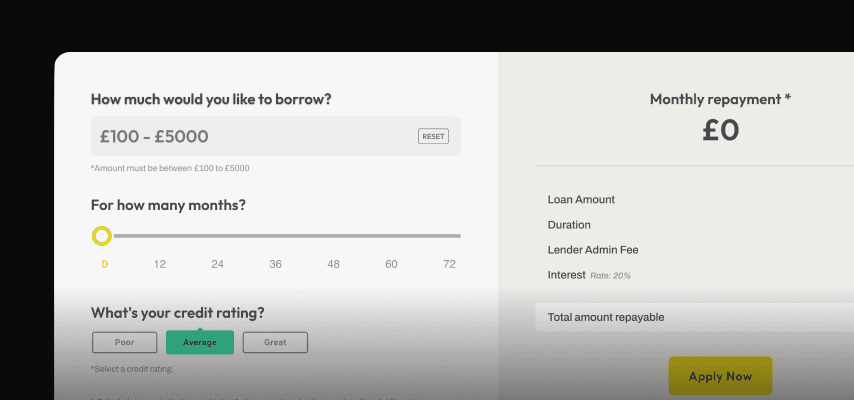
Real Loan Calculator
Add a fully functional, responsive, configurable loan calculator to your project.
Section
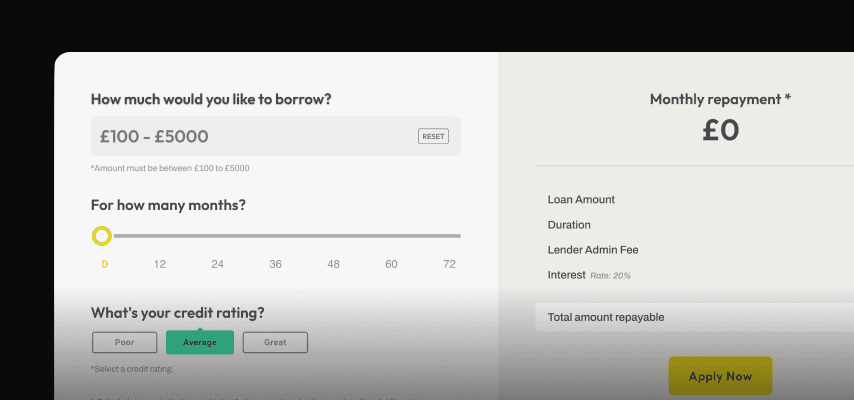
Real Loan Calculator
Add a fully functional, responsive, configurable loan calculator to your project.
Section
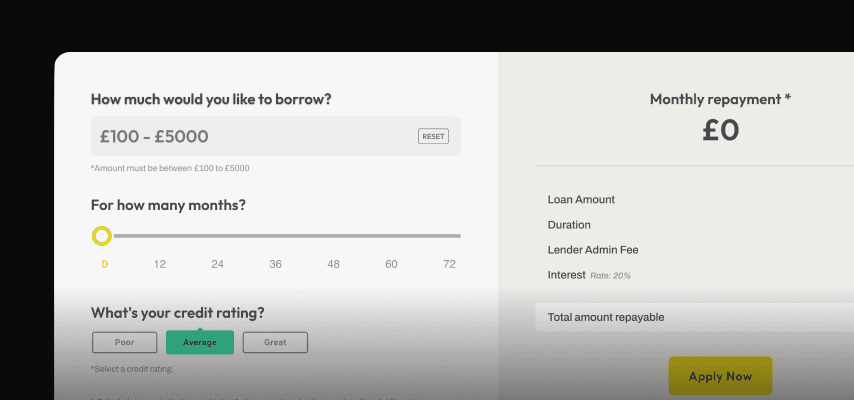
Real Loan Calculator
Add a fully functional, responsive, configurable loan calculator to your project.
Section
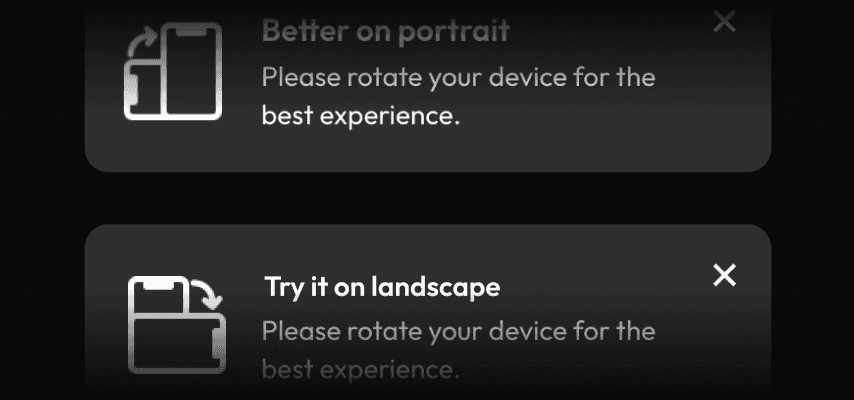
Mobile Rotation Alert
If your site works better in one orientation than another why not let your users know with this little component.
Code Component
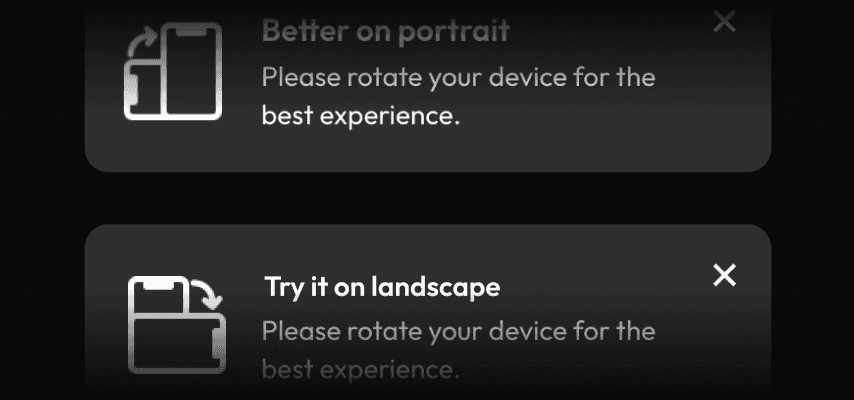
Mobile Rotation Alert
If your site works better in one orientation than another why not let your users know with this little component.
Code Component
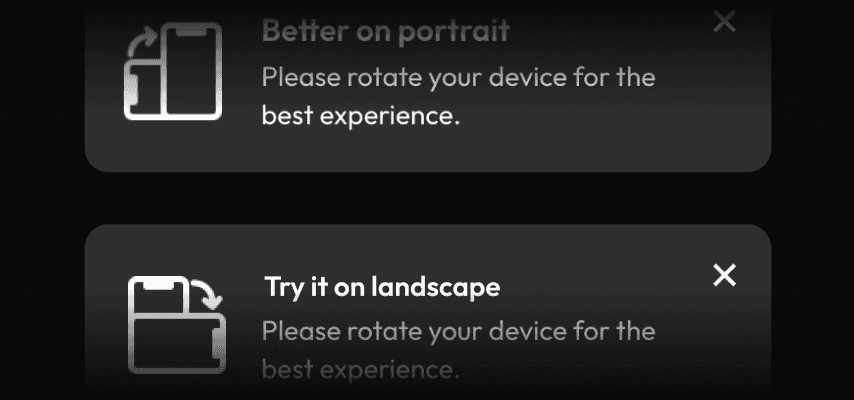
Mobile Rotation Alert
If your site works better in one orientation than another why not let your users know with this little component.
Code Component